일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
- 백엔드
- HTML
- 프론트엔드
- react
- 타입스크립트
- next.js
- 클라우드
- kubernetes
- 알고리즘
- 웹
- 컴퓨터공학
- CSS
- k8s
- TypeScript
- VUE
- 백준
- 리액트
- 자바스크립트
- 이더리움
- BFS
- 파이썬
- es6
- 쿠버네티스
- 이슈
- JavaScript
- 가상화
- 블록체인
- 솔리디티
- docker
- AWS
- Today
- Total
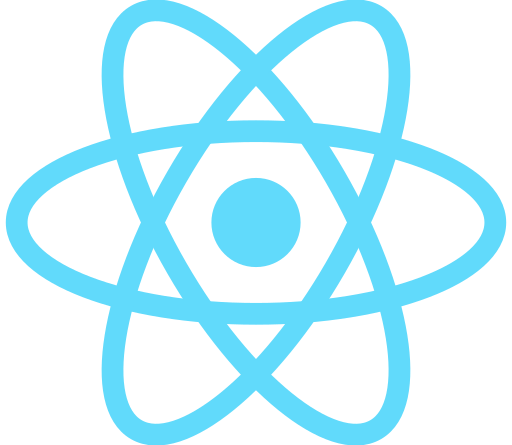
즐겁게, 코드
유틸리티 타입 - Partial, Required, Pick 사용하기 본문
타입스크립트에는 원시 타입 외에도 타입을 조작할 수 있는 유틸리티 타입이 존재합니다.
오늘은 여러 유틸리티 타입 중 Partial
, Required
, Pick
이라는 타입을 사용해 보겠습니다.
type userType = {
id: number;
email: string;
password: string;
firstname: string;
lastname: string;
};
여기 이름, 비밀번호, 이메일, 고유번호로 구성된 타입이 있습니다.
하지만 어떤 컴포넌트에서는 비밀번호가 필요하지 않을 수도 있고, 또다른 컴포넌트에서는 이메일이 필요하지 않을 수도 있습니다.
type userType = {
id?: number;
email?: string;
password?: string;
firstname?: string;
lastname?: string;
};
물론 이렇게 모든 속성을 옵셔널로 지정하면 원하는 속성만 포함된 타입을 사용할 수 있지만, 이러면 타입 검사가 느슨해짐으로써 결국 타입스크립트를 사용하는 의미가 퇴색되게 됩니다.
type userType = {
id: number;
email: string;
password: string;
firstname: string;
lastname: string;
};
type withoutPasswordUserType = {
id: number;
email: string;
firstname: string;
lastname: string;
}
type withoutEmailUserType = {
id: number;
password: string;
firstname: string;
lastname: string;
}
그러면 이렇게 원하는 요소들만 포함된 커스텀 타입을 필요할 때마다 만들어야만 할까요?
만약 속성이 10개, 20개 이런 식으로 많아지면 어떻게 할까요?
어쩌다보니 서론이 길어졌는데, 바로 이럴 때 유틸리티 타입을 활용할 수 있습니다.
유틸리티 타입 1 : Partial 타입 사용하기
type userType = {
id: number;
email: string;
password: string;
firstname: string;
lastname: string;
};
맨 처음 보았던 유저 타입입니다.
Partial
유틸리티 타입을 활용하면, 모든 요소를 옵셔널로 지정한 타입을 새로 생성할 수 있습니다.
type userType = {
id: number;
email: string;
password: string;
firstname: string;
lastname: string;
};
// Partial은 인자 타입의 모든 속성을 옵셔널로 변경한 타입입니다.
const user: Partial<userType> = {
id: "kimchanmin1";
// password: "12345",
email: "kimchanmin1@gmail.com";
firstname: "찬민";
lastname: "김";
}
유틸리티 타입 2 : Pick 타입 사용하기
pick
타입은 특정 요소만 포함된 타입을 생성합니다.
type userType = {
id: number;
email: string;
password: string;
firstname: string;
lastname: string;
};
// Pick은 첫 번째 인자로 받은 타입 중, 두 번째 인자로 주어진 속성만을 포함한 타입을 리턴합니다.
const user: Pick<userType, "id" | "firstname" | "lastname"> = {
id: "kimchanmin1";
firstname: "찬민";
lastname: "김";
}
유틸리티 타입 3 : Required 타입 사용하기
Required
타입은 Partial
타입처럼 모든 속성이 포함된 타입을 리턴하지만, 모든 속성을 필수로 요구합니다.
따라서 단독으로 사용할 기회는 많지 않을수도 있지만, Pick
등 다른 유틸리티 타입과 결합해 새로운 타입을 쉽게 생성할 수 있습니다.
const user = {id: 1, lastname: "김", firstname: "찬민"};
const user1: Required<Pick<userType, "id" | "lastname" | "firstname">> = user;
이처럼 타입스크립트에는 문자열, 숫자 등의 원시 자료형뿐만 아니라 기존 타입들을 가공하는 유틸리티 타입이라는 것이 존재함을 알 수 있었습니다.
오늘 다룬 Partial
, Pick
, Required
외에도 상황에 따라 사용할 수 있는 유틸리티 타입이 다양하니, 더 궁금하신 분들은 한번 공식 문서를 통해 확인해보시길 추천드리겠습니다. 😁
Documentation - Utility Types
Types which are globally included in TypeScript
www.typescriptlang.org
'🎨 프론트엔드 > Typescript' 카테고리의 다른 글
import type 구문으로 명시적으로 타입 불러오기 (0) | 2023.06.18 |
---|---|
배열아 꼼짝 마라! - readonly 타입 사용하기 (0) | 2021.12.26 |
타입스크립트에서 이벤트 객체 타입 지정하기 (2) | 2021.05.29 |
타입스크립트로 리액트 컴포넌트 구성해보기 (0) | 2021.05.21 |
tsc 옵션 제대로 사용하기 (0) | 2021.03.17 |