일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- JavaScript
- AWS
- HTML
- 파이썬
- 백준
- 클라우드
- 리액트
- 이더리움
- 백엔드
- 컴퓨터공학
- 웹
- CSS
- 이슈
- kubernetes
- VUE
- 타입스크립트
- es6
- BFS
- next.js
- 솔리디티
- 자바스크립트
- 알고리즘
- react
- 쿠버네티스
- 프론트엔드
- docker
- TypeScript
- 블록체인
- 가상화
- k8s
- Today
- Total
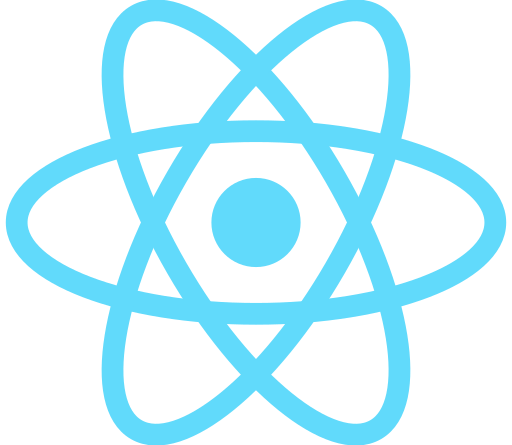
즐겁게, 코드
create-react-app과 Tailwind CSS 함께 사용하기 본문
Tailwind CSS가 요즘 새로운 CSS 프레임워크로 떠오르고 있습니다.
Tailwind CSS는 부트스트랩처럼 클래스명을 통해 전처리된 스타일을 적용할 수 있지만, 여기에 더해 tailwind.config.js
파일을 통해 다크 모드 설정, 추가할 플러그인 등 부트스트랩보다 훨씬 광범위하고 편리한 커스텀 기능을 제공합니다.
음... 사실 이렇게 말씀드리면 잘 와닿지 않으실 수도 있어 공식 문서의 예제를 하나 빌려보겠습니다.
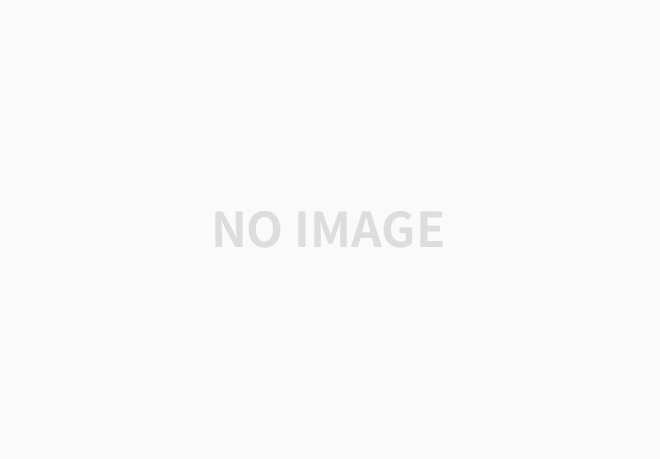
<div class="chat-notification">
<div class="chat-notification-logo-wrapper">
<img class="chat-notification-logo" src="/img/logo.svg" alt="ChitChat Logo">
</div>
<div class="chat-notification-content">
<h4 class="chat-notification-title">ChitChat</h4>
<p class="chat-notification-message">You have a new message!</p>
</div>
</div>
<style>
.chat-notification {
display: flex;
max-width: 24rem;
margin: 0 auto;
padding: 1.5rem;
border-radius: 0.5rem;
background-color: #fff;
box-shadow: 0 20px 25px -5px rgba(0, 0, 0, 0.1), 0 10px 10px -5px rgba(0, 0, 0, 0.04);
}
.chat-notification-logo-wrapper {
flex-shrink: 0;
}
.chat-notification-logo {
height: 3rem;
width: 3rem;
}
.chat-notification-content {
margin-left: 1.5rem;
padding-top: 0.25rem;
}
.chat-notification-title {
color: #1a202c;
font-size: 1.25rem;
line-height: 1.25;
}
.chat-notification-message {
color: #718096;
font-size: 1rem;
line-height: 1.5;
}
</style>
위 UI를 구현하기 위해서는 이렇게 장황한 CSS가 필요합니다.
만약 CSS에 익숙하지 않다면 여기서 더 헤매야만 하겠죠.
그러나 테일윈드를 활용하면, 이 장황한 코드가 놀랄 만큼 줄어들게 됩니다.
<div class="p-6 max-w-sm mx-auto bg-white rounded-xl shadow-md flex items-center space-x-4">
<div class="flex-shrink-0">
<img class="h-12 w-12" src="/img/logo.svg" alt="ChitChat Logo">
</div>
<div>
<div class="text-xl font-medium text-black">ChitChat</div>
<p class="text-gray-500">You have a new message!</p>
</div>
</div>
저도 사실 "왜 테일윈드 테일윈드 하는거지?" 하다가 이 예제를 보고 입을 싹 다물었는데요, 아무튼 간만에 부트스트랩처럼 쉽고 널리 사용할 수 있는 CSS 프레임워크가 등장했다는 것은 매우 반가운 소식입니다.
그럼 오늘은 리액트에서 테일윈드를 활용하기 위한 설정 방법을 정리해보도록 하겠습니다.
🛠 CRA로 구축한 리액트 프로젝트에 테일윈드 적용하기
create-react-app으로 리액트 프로젝트를 생성한 상태라고 가정해 보겠습니다.
1. tailwind 프레임워크 설치
이제 테일윈드를 적용하기 위한 의존성을 아래 명령어로 설치해 주세요.
npm install -D tailwindcss@npm:@tailwindcss/postcss7-compat postcss@^7 autoprefixer@^9
2. craco 설치 및 설정
cra는 기본적으로 PostCSS 속성을 덮어쓸 수 없도록 설정되어 있는데요, 이를 무시할 수 있게 해주는 craco 라이브러리를 설치합니다.
npm install @craco/craco
이후 리액트 스크립트의 설정을 덮어쓸 수 있도록 packages.json
의 스크립트 부분을 아래와 같이 바꿔줍니다.
{
// ...
"scripts": {
"start": "craco start",
"build": "craco build",
"test": "craco test",
"eject": "react-scripts eject"
},
}
이제 CRA의 postcss 속성을 테일윈드를 사용하도록 덮어쓸 차례입니다.
craco.config.js
를 프로젝트 최상단(src 바깥)에 생성한 후, 다음과 같이 본문을 작성합니다.
// craco.config.js
module.exports = {
style: {
postcss: {
plugins: [
require('tailwindcss'),
require('autoprefixer'),
],
},
},
}
3. tailwind 설정 파일 생성
tailwind.config.js
파일을 생성하기 위해 아래 명령어를 입력합니다.
npx tailwindcss init
그럼 아래와 같은 테일윈드 기본 설정 파일이 생성됩니다.
module.exports = {
purge: [],
darkMode: false, // or 'media' or 'class'
theme: {
extend: {},
},
variants: {
extend: {},
},
plugins: [],
}
만약 테일윈드가 자랑하는 극한의 용량 최적화를 사용하고 싶다면, purge 배열의 값을 다음과 같이 바꿔줍니다.
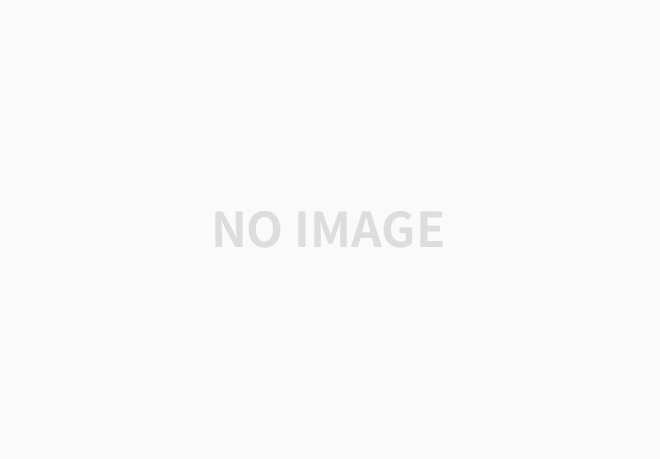
module.exports = {
// purge 배열을 변경해 줍니다.
purge: ['./src/**/*.{js,jsx,ts,tsx}', './public/index.html'],
darkMode: false, // or 'media' or 'class'
theme: {
extend: {},
},
variants: {
extend: {},
},
plugins: [],
}
4. 스타일에 반영하기
/* index.css 또는 루트 파일에 포함되는 css 파일 */
/* 특이하게도 @import 가 아닌 @tailwind 키워드로 모듈을 불러옵니다! */
@tailwind base;
@tailwind components;
@tailwind utilities;
위 코드를 app.js
또는 index.js
에 포함되는 루트 스타일 파일에 추가해주시면, 테일윈드를 사용할 준비가 모두 끝났습니다.
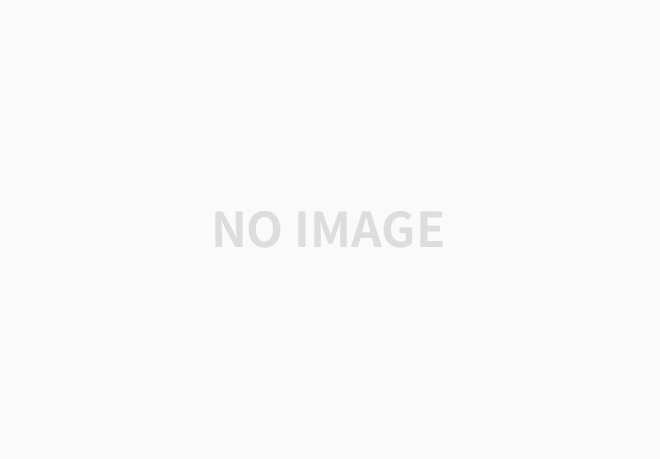
<div className="App">
<header className="text-gray-100 bg-blue-500 text-center p-5 align-middle">
redux-saga를 연습해 봅시다
</header>
<div className="pl-10 pt-10 text-xl">초기 데이터를 이곳에 보여줘야 합니다.</div>
<button className="p-3 border m-10 rounded-xl bg-blue-100" onClick={fetchData}>
데이터 불러오기
</button>
<Container memberList={memberList} />
</div>
제가 짧게 써본 소감은... 이건 진짜다?
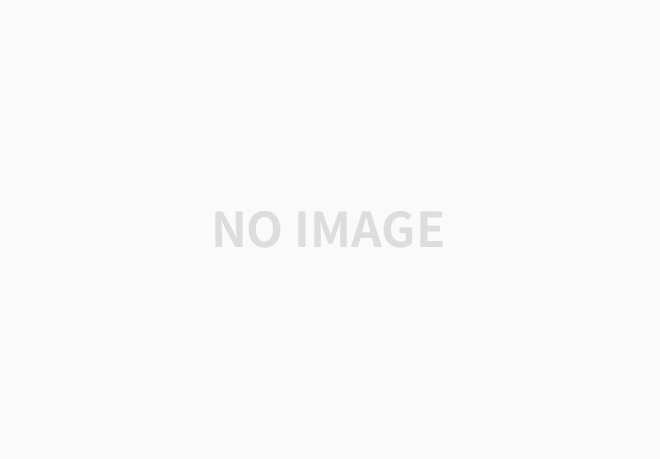
부트스트랩과 비교하자면 부트스트랩은 사용할수록 특유의 느낌을 숨기기 어려웠는데, 테일윈드는 단순히 CSS를 작성하기 쉽도록 하는 도구라고 느껴졌습니다. (위의 예제 및 다른 예제들에서도 볼 수 있듯, 테일윈드는 특유의 느낌이 따로 남지 않습니다.)
저는 지금까지 스타일링 방식을 컴포넌트별 폴더 안에 컴포넌트.jsx
와 컴포넌트.scss
를 매칭시켜 사용해왔는데, 앞으로는 스타일드 컴포넌트와 테일윈드를 주로 활용할 것 같습니다.
물론 도구를 선택하는건 여러분의 몫이겠지만, 저는 테일윈드가 충분히 배울만한 가치가 있다고 생각되네요! 😁
츄라이 츄라이!
'🎨 프론트엔드 > UI Library' 카테고리의 다른 글
Tailwind CSS : JIT 모드에 대하여 (0) | 2021.10.11 |
---|---|
Scss 파일에서 다른 스타일시트 불러오기 (0) | 2021.03.09 |
머터리얼 UI에서 미디어쿼리 사용하기 (2) | 2021.01.08 |