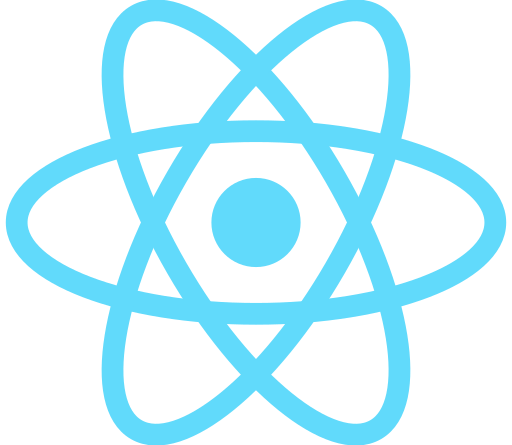
즐겁게, 코드
데이터 페칭 로직 우아하게 개선하기 본문
요즘에는 아마 많은 분들이 프라미스의 동작 순서를 직관적으로 표현하기 위해 async-await
문법을 사용하고 계실 텐데요, async-await
를 사용하는 것만으로도 어느 정도 코드가 읽기 편해지지만 여기서 더 우아한 로직을 작성하는 방법을 공유해보려 합니다.
const getStudentList = async () => {
const res = await fetch("http://localhost:5000/students");
return res.json();
};
const getGradeInfo = async () => {
const res = await fetch("http://localhost:5000/grades");
return res.json();
};
const fetchData = async () => {
try {
const studentList = await getStudentList("http://localhost:5000/students");
} catch (err) {
console.log("학생 정보를 불러오는데 실패했습니다.");
}
try {
const gradeList = await getGradeInfo("http://localhost:5000/grades");
} catch (err) {
console.log("성적 정보를 불러오는데 실패했습니다.");
}
// ... todo with studentList & gradeList
};
// 학생, 성적 정보를 비동기적으로 불러옵니다.
fetchData();
getStudentList
와 getGradeInfo
는 각각 학생 목록과 성적 정보를 불러오는 코드인데요, 여기에 예외처리 로직을 추가로 작성하다 보니 fetchData
함수 내부가 다소 복잡해 보이는 모습입니다.
그럼 한번 위 코드를 개선해 보겠습니다.
const getStudentList = async () => {
try {
const res = await fetch("http://localhost:5000/students");
const data = res.json();
return data;
} catch (err) {
console.log("학생 정보를 불러오는데 실패했습니다.");
return err;
}
};
const getGradeInfo = async () => {
try {
const res = await fetch("http://localhost:5000/grades");
const data = res.json();
return data;
} catch (err) {
console.log("성적 정보를 불러오는데 실패했습니다.");
return err;
}
};
const fetchData = async () => {
const studentList = await getStudentList("http://localhost:5000/students");
const gradeList = await getGradeInfo("http://localhost:5000/grades");
// ... todo with studentList & gradeList
};
// 학생, 성적 정보를 비동기적으로 불러옵니다.
fetchData();
getStudentList
와 getGradeInfo
내에서 예외처리를 수행함으로써 둘을 감싸고 있는 fetchData
가 보다 깔끔해지긴 했지만, 이러면 studentList
에 데이터가 들어올지 에러 객체가 들어올지 예측할 수 없어 로직을 추가로 작성해야 한다는 단점이 존재합니다.
그래서 이렇게 작성하는 방법이 있습니다.
const getStudentList = async () => {
try {
const res = await fetch("http://localhost:5000/students");
const data = res.json();
return [data, null];
} catch (err) {
return [null, err];
}
};
const getGradeInfo = async () => {
try {
const res = await fetch("http://localhost:5000/grades");
const data = res.json();
return [data, null];
} catch (err) {
return [null, err];
}
};
const fetchData = async () => {
const [studentList, errorOnStudent] = await getStudentList("http://localhost:5000/students");
const [gradeList, errorOnGrade] = await getGradeInfo("http://localhost:5000/grades");
// ... todo with studentList & gradeList
};
// 학생, 성적 정보를 비동기적으로 불러옵니다.
fetchData();
바로 배열의 분해 할당 문법을 활용해 첫 번째 원소는 데이터, 두 번째 원소에는 에러 객체가 담기도록 한 것인데요, 이렇게 함으로써 외부 코드의 흐름을 매끄럽게 개선함과 동시에 페칭 함수들이 데이터를 반환하는지 에러 객체를 반환하는지도 명확하게 판별할 수 있게 됩니다.
이런 사소한 개선이 좋은 코드를 작성하는데 도움이 되길 바라며, 이번 한 주도 행복하시길 바라겠습니다 :>
반응형
'💬 언어 > Javascript' 카테고리의 다른 글
얼어붙어라! - 객체 불변화하기 (0) | 2021.08.26 |
---|---|
keys, values, entries 메서드 사용하기 (0) | 2021.08.15 |
너! 배열이 되어라! - Array.from() 메서드 사용하기 (1) | 2021.05.29 |
제너레이터 사용하기 - 지연 평가 (lazy evaluation) (0) | 2021.05.03 |
nullish coalescing 연산자로 널 참조 방지하기 (0) | 2021.05.02 |
Comments
소소한 팁 : 광고를 눌러주시면, 제가 뮤지컬을 마음껏 보러다닐 수 있어요!
와!! 바로 눌러야겠네요! 😆