일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 | 31 |
Tags
- 이더리움
- VUE
- next.js
- HTML
- 컴퓨터공학
- 이슈
- 블록체인
- CSS
- 리액트
- 프론트엔드
- docker
- TypeScript
- 백엔드
- es6
- 클라우드
- 웹
- JavaScript
- kubernetes
- k8s
- react
- 알고리즘
- 쿠버네티스
- 자바스크립트
- 파이썬
- BFS
- AWS
- 솔리디티
- 가상화
- 타입스크립트
- 백준
Archives
- Today
- Total
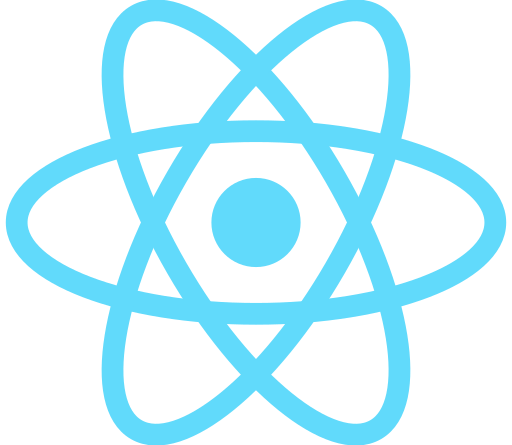
즐겁게, 코드
솔리디티 - 07. 컨트랙트의 소유권 본문
1. 컨트랙트의 소유권
pragma solidity "^0.4.19";
contract myContract {
function sendEther(address _address, uint _amount) external {
send(_address, _amount);
}
}
이더(돈)를 보내는 sendEther 라는 함수가 컨트랙트에 존재하는 상태입니다.
다만 sendEther는 external
로 선언되어 누구나 호출할 수 있는 상태인데요, 컨트랙트의 소유자만 특정 함수에 접근할 수 있도록 하기 위해 Ownable 이라는 라이브러리를 활용합니다.
[Ownable.sol 라이브러리 코드 전문]
/**
* @title Ownable
* @dev The Ownable contract has an owner address, and provides basic authorization control
* functions, this simplifies the implementation of "user permissions".
*/
contract Ownable {
address public owner;
event OwnershipTransferred(address indexed previousOwner, address indexed newOwner);
/**
* @dev The Ownable constructor sets the original `owner` of the contract to the sender
* account.
*/
function Ownable() public {
owner = msg.sender;
}
/**
* @dev Throws if called by any account other than the owner.
*/
modifier onlyOwner() {
require(msg.sender == owner);
_;
}
/**
* @dev Allows the current owner to transfer control of the contract to a newOwner.
* @param newOwner The address to transfer ownership to.
*/
function transferOwnership(address newOwner) public onlyOwner {
require(newOwner != address(0));
OwnershipTransferred(owner, newOwner);
owner = newOwner;
}
}
Ownable 라이브러리를 간단하게 설명해보겠습니다.
Ownable
함수는 생성자로써 컨트랙트가 생성될 때 owner 변수를 현재 호출자의 주소로 초기화합니다.onlyOwner
함수는 함수 제어자로써, 제어자가 작용된 함수가 실행되기 전에 제어자를 호출합니다.
function Ownable() public {
owner = msg.sender;
}
modifier onlyOwner() {
require(msg.sender == owner);
_;
}
이제 본격적으로 Ownable을 사용해 보겠습니다.
먼저 Ownable.sol을 불러온 뒤, 컨트랙트가 Ownable을 상속받도록 합니다.
pragma solidity "^0.4.19";
import "./ownable.sol"
contract myContract is Ownable {
function sendEther(address _address, uint _amount) external {
send(_address, _amount);
}
}
이제 onlyOwner
라는 함수 제어자를 활용합니다.
function sendEther(address _address, uint _amount) external onlyOwner {
// onlyOwner 제어자의 본문이 함수 본문에 포함된 상태라고 보시면 됩니다.
// require(msg.sender == owner);
send(_address, _amount);
}
이제 이더를 전송하는 sendEther
함수는 호출자가 소유권자와 동일한지 매번 자동으로 검사하게 되어, 컨트랙트의 소유권자만이 안전하게 이더를 전송할 수 있게 됩니다.
반응형
'💬 언어 > Solidity' 카테고리의 다른 글
이더리움의 표준, ERC-20 인터페이스와 구현 (2) | 2021.04.22 |
---|---|
솔리디티 - 08. 가스 (Gas), 가스 최적화 기법 (0) | 2021.04.19 |
솔리디티 - 06. 상속과 인터페이스 (0) | 2021.04.16 |
솔리디티 - 05. Storage와 Memory (0) | 2021.04.16 |
솔리디티 - 04. 매핑과 msg.sender (0) | 2021.04.16 |
Comments
소소한 팁 : 광고를 눌러주시면, 제가 뮤지컬을 마음껏 보러다닐 수 있어요!
와!! 바로 눌러야겠네요! 😆